Introduction
In the field of web development, rigorous testing is important to ensure the reliable functionality of the website or the web application. Selenium, a preeminent tool for validating browser behaviour, becomes substantially more powerful when integrated with the Page Object Model (POM). This article explains why the Page Object Model is considered a superior approach in Selenium projects and provides a comprehensive guide to implementing POM in your project. So, let's get started on how POM enhances Selenium’s capabilities and how its utilisation can augment your testing procedures' precision, efficiency, and reliability.
Understanding the Page Object Model (POM)
The Page Object Model (POM) is like a blueprint that makes Selenium code much easier to handle, organise, and work with. It is known to reduce all the clutter in the code and keep it organised according to its respective pages. Let us imagine each webpage as a character in a story, and POM assigns each character a class to describe what it looks like and can do. This method makes writing test scripts easy; the code looks clean, and any changes made can be easy to handle.
Here is what the screen should look like while implementing POM.
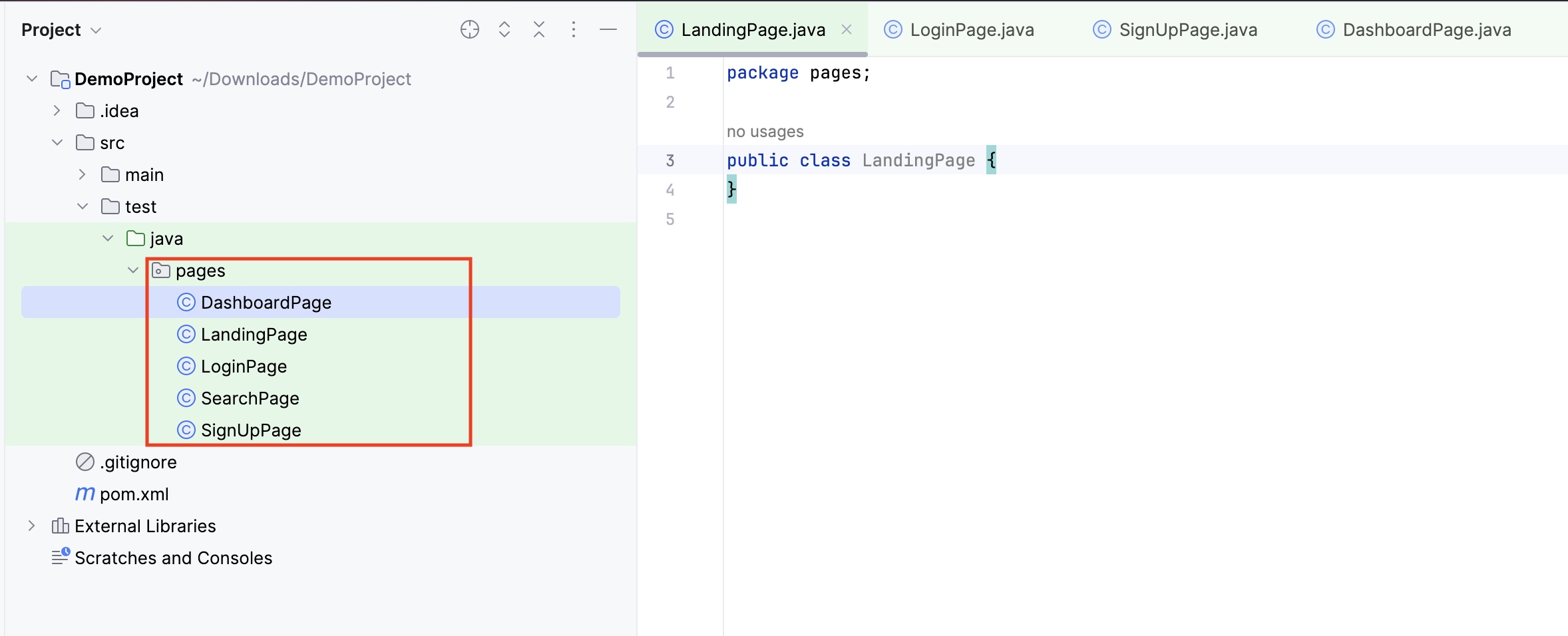
The image above shows the individual classes that have been created for various pages, such as Dashboard Page
, Landing Page
, Login Page
, Signup Page
, and Search Page
. Each class consists of code specific to its respective page, which helps organise the code and avoid mess on a single page.
Now, let's discuss why integrating the Page Object Model in Selenium is a great idea and how it makes testing easier.
Advantages of Page Object Model(POM) :
- Better Organisation: POM will make finding your code and keeping it well-organised easier. It's like putting the books in the library in the order they belong so they can be easily retrieved whenever they are used.
- Reuse of Code: The POM feature will allow you to write the same test codes, which will, in turn, give you two benefits: saving time and effort simultaneously. It feels like having a recipe box containing different dishes you can prepare using the ingredients you have without cooking from scratch every time you want to prepare them.
- Easier to Understand: POM divides code to reduce page code and simplify figuring out and adjusting. It’s like dividing a complex job into small, easily manageable bits.
- Simplified Error Detection: POM code is organised logically, so any errors noted will be easily spotted. This is equivalent to having labelled folders for different files; you always know where to check if something is wrong.
- Easier Maintenance: If you want to change some of the page elements, POM clearly shows you the necessary adjustments. It allows you to speed up the update process without hampering other functions.
Implementing the Page Object Model (POM)
- Create Page Classes: First, identify your application's individual web pages. For each web page, a dedicated class encapsulating the elements and actions relevant to that page should be created to ensure a clear separation.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
public class HomePage {
private WebDriver driver;
private By searchBox = By.id("search_box");
private By searchButton = By.id("search_button");
public HomePage(WebDriver driver) {
this.driver = driver;
}
public void searchForProduct(String query) {
driver.findElement(searchBox).sendKeys(query);
driver.findElement(searchButton).click();
}
}
Code snippets of Homepage Class
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
public class CartPage {
private WebDriver driver;
private By checkoutButton = By.id("checkout_button");
public CartPage(WebDriver driver) {
this.driver = driver;
}
public void proceedToCheckout() {
driver.findElement(checkoutButton).click();
}
}
Code snippets of Cart Page Class
The code contains two classes: one for the Homepage of a website and another for the cart page. Each class has its own methods to deal with elements like clicking buttons or typing in text boxes on their respective pages.
- Craft Test Scripts: After creating each page class, we incorporate a separate test script for each class. Within these scripts, the methods defined in the page classes should be used to interact with the corresponding web elements on the pages.
public class TestHomePage {
private WebDriver driver;
private HomePage homePage;
@Before
public void setUp() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
driver = new ChromeDriver();
driver.get("http://example.com");
homePage = new HomePage(driver);
}
@Test
public void testSearchProduct() {
homePage.searchForProduct("laptop");
// Add assertions to verify search results
}
@After
public void tearDown() {
driver.quit();
}
}
Test script of Homepage
This test script verifies functionality on the Home Page. It sets up the web driver, navigates to the Home Page, performs a search operation, and can include assertions to verify the search results.
public class TestCartPage {
private WebDriver driver;
private CartPage cartPage;
@Before
public void setUp() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
driver = new ChromeDriver();
driver.get("http://example.com/cart");
cartPage = new CartPage(driver);
}
@Test
public void testProceedToCheckout() {
cartPage.proceedToCheckout();
}
@After
public void tearDown() {
driver.quit();
}
}
Test script of Cart Page
This test script verifies functionality on the Cart Page. It sets up the Web driver, navigates to the Cart Page, performs an action (proceeding to checkout), and can include assertions to verify the action.
- Utilise Page Objects: We normally interact directly with the web elements. We can use page classes as objects instead of directly interacting with web elements. This approach promotes re-usability and maintains a clear view between test logic and page-specific functionality.
- Employ Page-factory: Selenium's Page-factory model can dynamically initialise web elements within the page classes. This helps simplify the initialisation process and improve the code's readability.
- Manage Locators Separately: A separate file should be created for storing element locators. Separating locators from the code base makes it easier to update them as needed without modifying the entire test logic.
You can store locators in a separate file like this:
import org.openqa.selenium.By;
public class HomePageLocators {
public static final By SEARCH_BOX = By.id("search_box");
public static final By SEARCH_BUTTON = By.id("search_button");
}
public class ProductPageLocators {
public static final By ADD_TO_CART_BUTTON = By.id("add_to_cart_button");
}
public class CartPageLocators {
public static final By CHECKOUT_BUTTON = By.id("checkout_button");
}
Code snippets of the Locator file
- Separate Page Logic: Logic and Method should be kept with each page within its respective page classes only. This ensures that each page's functionality is located in its respective page classes and can be easily searched, maintained, or updated without affecting other parts of the code-base.
- Verify using Assertions: Implement assertions within the page classes to verify that the pages behave as expected. Assertions provide a mechanism for validating the correctness of page elements, ensuring that the tests accurately reflect the behaviour.
Conclusion
We can conclude that the POM model in Selenium makes the pathway for the testing process a lot easier and more organised. The way it breaks down the code for each webpage separately makes it easier for its users to understand and manage their projects efficiently. POM helps create a cleaner testing procedure by focusing on each web page's distinct parts. It is almost like organising your files into different folders on your PC, making it easier to find and update them when required without messing up everything else.
Thank you for reading this article. Please consider subscribing for more such articles.