Hello developer! After getting introduced to a few GUI Elements in my previous blog, today we will learn how to create a full GUI Drop Down Menu.
After going through this tutorial process, we will be able to get the result shown below.
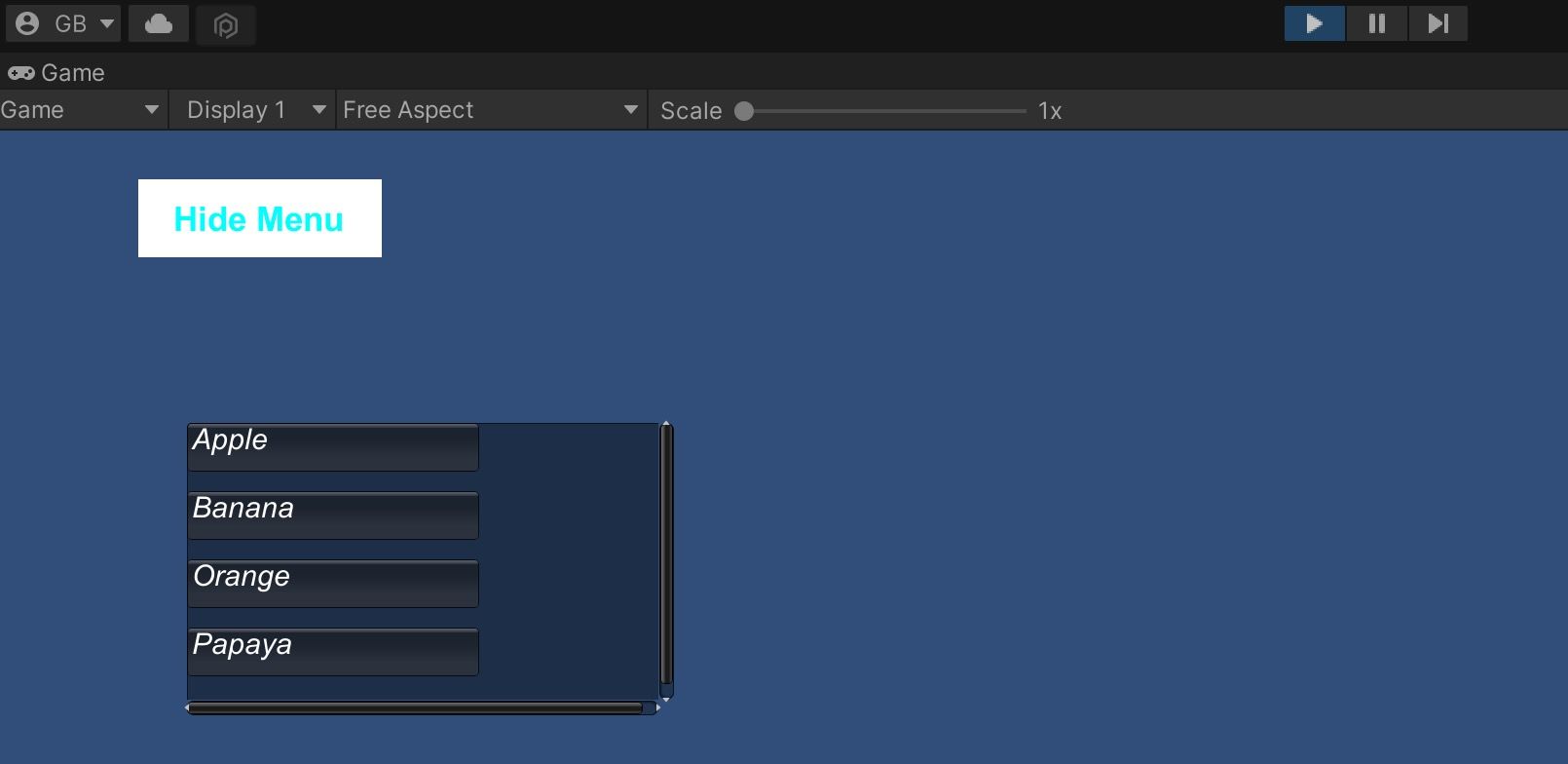
Our Drop Down Menu will consist of 3 GUI Elements.
- GUI Buttons: GUI Buttons will be used as Main Menu Button and fruit option buttons in the ScrollView.
- GUI ScrollView: GUI ScrollView will allow our element to have a horizontal and vertical Scroll Bar and fruits options to be selected.
- GUI Box: a GUI Box will be used as a background image to the ScrollView.
So, let's begin!
Creating Variables
We will create different useful variables first. Their uses are described in the comments of the code snippet below.
//Keeps the slider value from SliderView.
private Vector2 scrollPosition;
//List to show as selecting options.
private string[] fruitsList = {
"Apple",
"Banana",
"Orange",
"Papaya"
};
//Current Index of the selected fruit.
int currentFruitIndex;
//Boolean variable used to show and hide menu options.
private bool isShowOpenMenu;
//String value to update Menu Button click.
private string showMenuBtnText = "Show Menu";
///_scrollViewPositionRect provides position of ScrollView from first two parameters as x and y
///and provides width and Height of ScrollView from following two parameters as width and height.
private Rect _scrollViewPositionRect = new Rect(200, 300, 500, 300);
private float heightOfBtn = 50,
distanceBetweenEachBtn = 20;
//Image to assign to Menu Button.
[SerializeField] public Texture2D menuBtnImage;
///For the slider in scroll view to be visible scrollViewViewRect's width and height should be greater than _scrollViewPositionRect
///Because obviously if the view is smaller we would not need the slider.
private Rect _scrollViewViewRect = new Rect(0, 0, 501, 301);
//boxTopLeftPositionRect places Box at top left corner and reducing its height and width to half of GUI ScrollView height and width respectively.
private Rect boxTopLeftPositionRect = new Rect(0, 0, 500 / 2, 300 / 2),
boxTopRightPositionRect = new Rect(500 / 2, 0, 500 / 2, 300 / 2),
boxBottomLeftPositionRect = new Rect(0, 300 / 2, 500 / 2, 300 / 2),
boxBottomRightPositionRect = new Rect(500 / 2, 300 / 2, 500 / 2, 300 / 2)
//boxFullScreenViewRect has same width and height as _scrollViewPositionRect so it would be visible where ScrollView is visible.
,
boxFullScreenViewRect = new Rect(0, 0, 500, 300);
GUIStyle _menuBtnStyle = new GUIStyle(),
//GUI Style for Menu Button.
selectedItemDisplayStyle = new GUIStyle(),
//GUI style for output text.
_optionsBtnStyle = new GUIStyle(); //GUI style for options in drop down menu.
GUI Styling
We don't just want to create a drop-down menu but also present it beautifully. So, we can create different GUI Styles and define them.
void DefineStyles() {
//Customizing Menu Button properties.
_menuBtnStyle.fontSize = 35;
_menuBtnStyle.fontStyle = FontStyle.Bold;
_menuBtnStyle.alignment = TextAnchor.MiddleCenter;
_menuBtnStyle.normal.textColor = Color.cyan;
_menuBtnStyle.normal.background = menuBtnImage;
//Customizing output text properties.
selectedItemDisplayStyle.fontSize = 20;
selectedItemDisplayStyle.normal.textColor = Color.green;
//Customizing options properties.
_otionsBtnStyle.fontSize = 30;
_otionsBtnStyle.fontStyle = FontStyle.Italic;
_otionsBtnStyle.normal.textColor = Color.white;
}
Showing/Hiding Menu
We will create a button that will show and hide the drop-down menu.
if (GUI.Button(new Rect(150, 50, 250, 80), showMenuBtnText, _menuBtnStyle))
{
//Changing states for menu btn clicked.
if (!isShowOpenMenu)
{
isShowOpenMenu = true;
showMenuBtnText = "Hide Menu";
}
else
{
isShowOpenMenu = false;
showMenuBtnText = "Show Menu";
}
}
Now, let's write the code to show the drop-down menu when the button is pressed. In this step, we will be creating a ScrollView. We can set its dimensions by assigning _scrollViewPositionRect
and _scrollViewViewRect
. Also, let's create the GUI Box in this step.
//Enabling menu options and starting Scroll View.
scrollPosition = GUI.BeginScrollView(_scrollViewPositionRect, scrollPosition, _scrollViewViewRect);
//Creating GUI Box to give as a background image .
GUI.Box(boxFullScreenViewRect, "");
Filling Up Menu
Now it's time to create the fruit option buttons and text for them. We will create buttons, set their position, and add a GUI label as the text view for those buttons.
for (int i = 0; i < 4; i++) {
if (GUI.Button(new Rect(0, i * (heightOfBtn + distanceBetweenEachBtn), 300, heightOfBtn), "")) {
//Following statements will execute on Button Click
isShowOpenMenu = false;
showMenuBtnText = "Show Menu";
currentFruitIndex = i;
}
//Setting text value and position such that it looks like the text of button.
//But actual text of button is null in the place where button is defined.
GUI.Label(new Rect(5, i * (heightOfBtn + distanceBetweenEachBtn), 300, heightOfBtn), fruitsList[i], _otionsBtnStyle);
}
Final Script
Let's see the whole script and what it looks like.
public class DropDownGUi: MonoBehaviour {
//Keeps the slider value from SliderView.
private Vector2 scrollPosition;
//List to show as selecting options.
private string[] fruitsList = {
"Apple",
"Banana",
"Orange",
"Papaya"
};
//Current Index of the selected fruit.
int currentFruitIndex;
//Boolean variable used to show and hide menu options.
private bool isShowOpenMenu;
//String value to update Menu Button click.
private string showMenuBtnText = "Show Menu";
///_scrollViewPositionRect provides position of ScrollView from first two parameters as x and y
///and provides width and Height of ScrollView from following two parameters as width and height.
private Rect _scrollViewPositionRect = new Rect(200, 300, 500, 300);
private float heightOfBtn = 50,
distanceBetweenEachBtn = 20;
//Image to assign to Menu Button.
[SerializeField] public Texture2D menuBtnImage;
///For the slider in scroll view to be visible scrollViewViewRect's width and height should be greater than _scrollViewPositionRect
///Because obviously if the view is smaller why would we need the slider.
private Rect _scrollViewViewRect = new Rect(0, 0, 501, 301);
//boxTopLeftPositionRect places Box at top left corner and reducing its height and width to half of GUI ScrollView height and width respectively.
private Rect boxTopLeftPositionRect = new Rect(0, 0, 500 / 2, 300 / 2),
boxTopRightPositionRect = new Rect(500 / 2, 0, 500 / 2, 300 / 2),
boxBottomLeftPositionRect = new Rect(0, 300 / 2, 500 / 2, 300 / 2),
boxBottomRightPositionRect = new Rect(500 / 2, 300 / 2, 500 / 2, 300 / 2)
//boxFullScreenViewRect has same width and height as _scrollViewPositionRect so it would be visible where ScrollView is visible.
,
boxFullScreenViewRect = new Rect(0, 0, 500, 300);
GUIStyle _menuBtnStyle = new GUIStyle(), //GUI Style for Menu Button.
selectedItemDisplayStyle = new GUIStyle(), //GUI style for output text.
_optionsBtnStyle = new GUIStyle(); //GUI style for options in drop down menu.
void Start() {
DefineStyles();
}
///This function defines GUI Styles to define properties related to font and dimensions.
void DefineStyles() {
//Customizing Menu Button properties.
_menuBtnStyle.fontSize = 35;
_menuBtnStyle.fontStyle = FontStyle.Bold;
_menuBtnStyle.alignment = TextAnchor.MiddleCenter;
_menuBtnStyle.normal.textColor = Color.cyan;
_menuBtnStyle.normal.background = menuBtnImage;
//Customizing output text properties.
selectedItemDisplayStyle.fontSize = 20;
selectedItemDisplayStyle.normal.textColor = Color.green;
//Customizing options properties.
_otionsBtnStyle.fontSize = 30;
_otionsBtnStyle.fontStyle = FontStyle.Italic;
_otionsBtnStyle.normal.textColor = Color.white;
}
void OnGUI() {
ShowGui();
}
void ShowGui() {
if (GUI.Button(new Rect(150, 50, 250, 80), showMenuBtnText, _menuBtnStyle)) {
//Changing states for menu btn clicked.
if (!isShowOpenMenu) {
isShowOpenMenu = true;
showMenuBtnText = "Hide Menu";
}
else {
isShowOpenMenu = false;
showMenuBtnText = "Show Menu";
}
}
if (isShowOpenMenu) {
//Enabling menu options and starting Scroll View.
scrollPosition = GUI.BeginScrollView(_scrollViewPositionRect, scrollPosition, _scrollViewViewRect);
//Creating GUI Box to give as a background image .
GUI.Box(boxFullScreenViewRect, "");
for (int i = 0; i < 4; i++) {
if (GUI.Button(new Rect(0, i * (heightOfBtn + distanceBetweenEachBtn), 300, heightOfBtn), "")) {
//Following statements will execute on Button Click
isShowOpenMenu = false;
showMenuBtnText = "Show Menu";
currentFruitIndex = i;
}
//Setting text value and position such that it looks like the text of button.
//But actual text of button is null in the place where button is defined.
GUI.Label(new Rect(5, i * (heightOfBtn + distanceBetweenEachBtn), 300, heightOfBtn), fruitsList[i], _otionsBtnStyle);
}
//Ending Scroll View.
GUI.EndScrollView();
}
else {
//This label is output text which is to show result of options clicked.
GUI.Label(new Rect(30, 50, 300, 25), fruitsList[currentFruitIndex], selectedItemDisplayStyle);
}
}
}
Finally, assign the Menu Button Image to anything of your choice in the inspector.
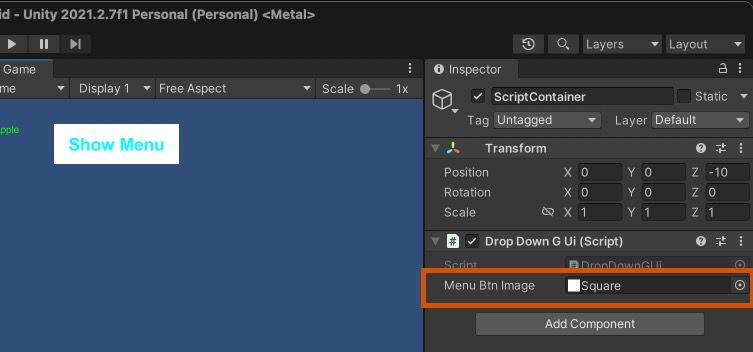
You should see the drop-down menu we just designed.
Thank you for reading this post till the end and I hope you have learned a new thing today!