Pre-Requisites
- Docker Environment
- Fundamental knowledge of Prisma ORM
Introduction to Prisma
At its core, Prisma is architected with a meticulous emphasis on performance and developer productivity. Leveraging the power of GraphQL, it seamlessly integrates with modern programming languages, offering a declarative approach to interact with databases. This abstraction layer fosters a symbiotic relationship between the application and the database, circumventing the complexities of traditional SQL queries while preserving the integrity of the underlying data model.
One of Prisma's hallmark features is its robust type safety mechanism. By leveraging the type systems inherent to programming languages like TypeScript, Prisma provides compile-time guarantees, mitigating the risk of runtime errors associated with raw SQL queries. This enhances code reliability and streamlines the development process by enabling comprehensive IDE support and intelligent auto-completion.
Furthermore, Prisma's schema-first approach empowers developers to define their data models using a concise and expressive syntax. This schema is a single source of truth, fostering team collaboration and facilitating seamless integration with version control systems. The ability to synchronize changes effortlessly between the application and the database ensures consistency and eliminates the potential for data schema drift.
Exposing Prisma Metrics
We don't need to go further than Prisma itself to expose Prisma Metrics. It can be simply enabled by adding a config line to the schema file
of Prisma. Currently, the feature is in preview but may soon be provided as a stable feature out of the box.
In the schema file
inside the generator block
, just add the previewFeatures line
as shown in the code block below.
generator client {
provider = "prisma-client-js"
previewFeatures = ["metrics"]
}
In the application that uses Prisma, create an endpoint, e.g. $BASEURL/db-
metrics, with the following code.
const metrics = await prisma.$metrics.prometheus()
console.log(metrics)
When your application is running, if you visit the db-metrics URL
, you will find a list of metrics Prometheus can consume.
For more info, visit the Prisma Documentation Site:
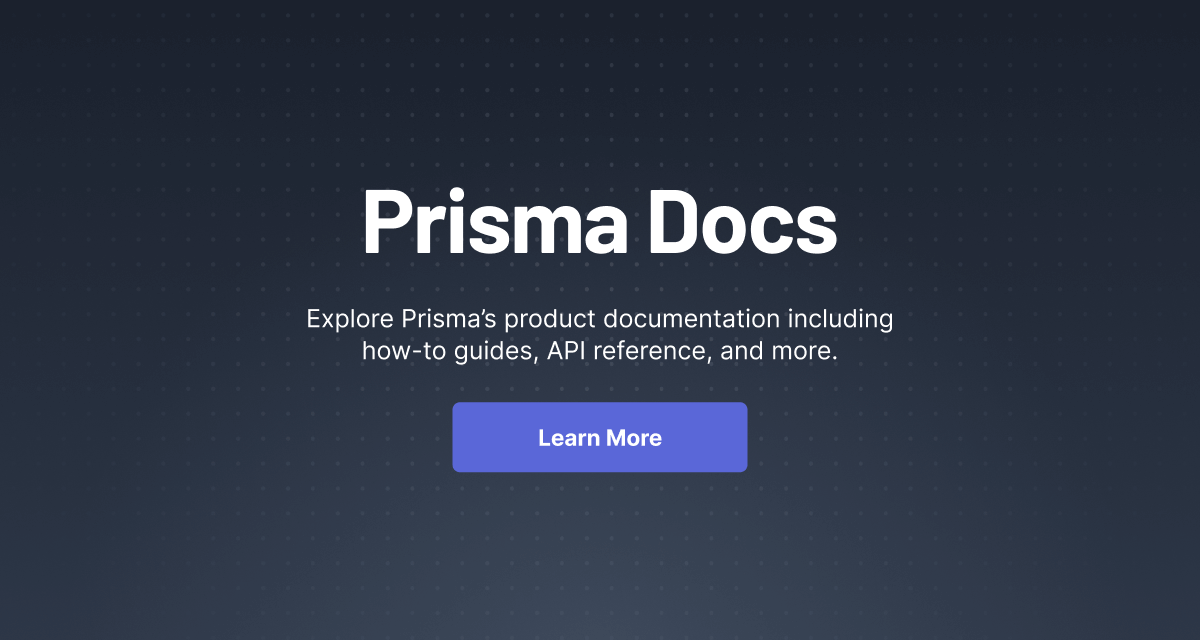
Now that our application and Prisma are ready to provide us with the metrics, we will set up Prometheus and Grafana in the next step, which will enable us to ingest and visualize the Prisma Metrics data.
Grafana & Prometheus Setup
Prometheus and Grafana collectively represent a formidable duo in the realm of monitoring and observability, serving as indispensable tools for top engineers striving to maintain the health, performance, and reliability of complex systems.
Prometheus, a time-series database and monitoring system, embodies the core tenets of scalability, reliability, and extensibility. Engineered with a distributed architecture in mind, Prometheus excels in collecting and aggregating metrics from diverse sources across dynamic, ephemeral environments. Its pull-based model and efficient storage and querying capabilities ensure minimal overhead and optimal performance, even at scale.
Complementing Prometheus, Grafana emerges as a visualization powerhouse, enabling engineers to transform raw metrics into actionable insights through rich, interactive dashboards. Grafana's intuitive user interface, visualization options, and templating capabilities empower engineers to craft bespoke dashboards tailored to their specific use cases and workflows.
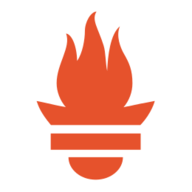
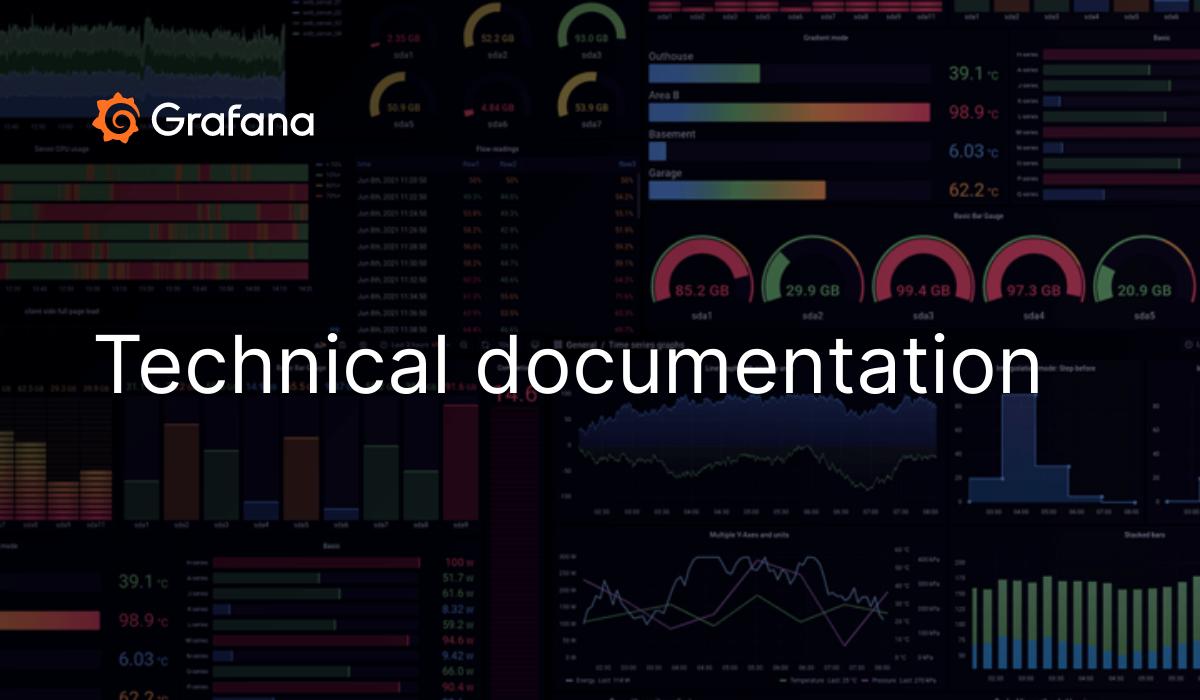
Setup
We will use docker compose
to set up the Prometheus and Grafana stack.
Save the file below as docker-compose.yml
.
version: "3.9"
name: prometheus-grafana
services:
prometheus:
image: prom/prometheus:latest
ports:
- 9000:9000
volumes:
- $PWD/prometheus.yml:/etc/prometheus/prometheus.yml
networks:
- promgraf
grafana:
image: grafana/grafana:latest
ports:
- 8000:8000
networks:
- promgraf
networks:
promgraf:
name: promgraf
attachable: true
Docker Compose for Prometheus and Grafana
Save the following two files as prometheus.yml
.
scrape_configs:
- job_name: 'app-metrics-prisma'
scrape_interval: 2s
static_configs:
- targets: ['host.docker.internal:3000']
labels:
service: 'app-metrics-prisma'
group: 'testing'
Prometheus.conf
Now, we will start them using the command,
docker compose -f docker-compose.yml up -d
The prometheus.yml
file already has the source of the metrics in it and has been provided with the time interval for scraping the endpoint. It can be configured further from the dashboard at localhost:9000
.
Grafana can be further configured at localhost:8000
. You can browse more templates, use them, and perform administrative tasks like user creation from the dashboard.
Conclusion
In this way, we learned about Prisma, Prometheus and Grafana. Learned to setup a metrics endpoint and visualize the exposed data in Grafana. You can explore more regarding this on the Prisma docs website or other related blogs.
Thank you for reading, please comment below of you have any queries. I try to update blogs periodically to ensure legibility.