Do you want your character to have dynamic movement when falling down? Well, ragdoll physics does that very easily. Setting up rag dolls in unity is pretty simple and I will show you how you can enable and disable them at run time. But before we dive into code to enable or disable ragdoll, we need to do the initial setup.
Setting Up Ragdoll :
- Select your character of choice and drag it into the scene.
- Add a plane below the character to stop the character from falling endlessly.
- To create the ragdoll of the current character, you need to first select it and right-click to open the ragdoll menu.
steps: right-click character -> 3D object -> ragdoll
- Now you need to assign bone to transform to the respective field in ragdoll wizard, here is an example of how you can do it. (Note: your bone name and ragdoll wizards naming conventions may not match)
- After everything has been set up, press create to create a ragdoll character
- You will need to adjust colliders in each bone if it is too big or small.
- After this, your ragdoll is ready to be used, but right now you cannot enable or disable it at runtime.
Here is the visual representation of how you can do all these
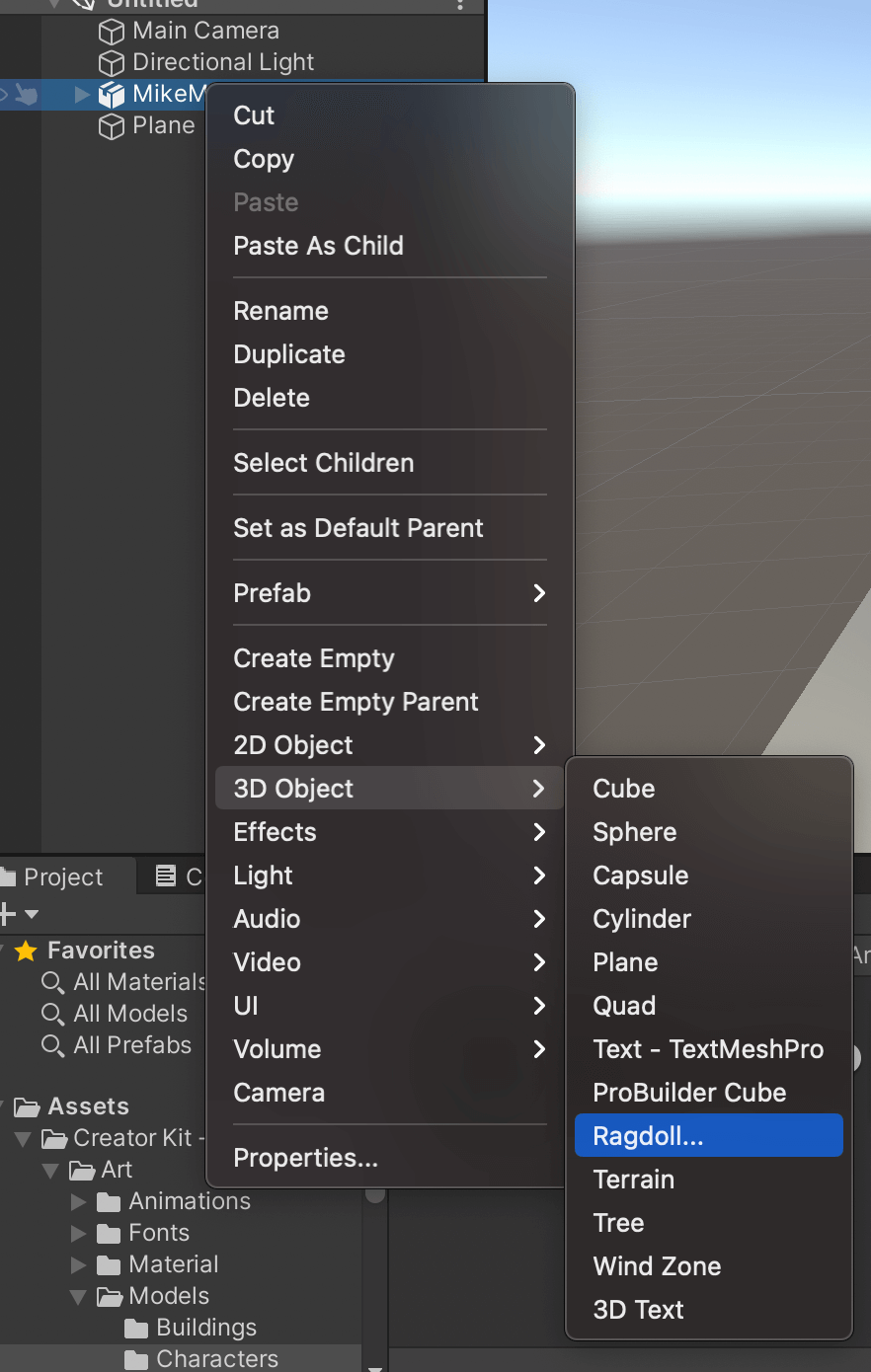
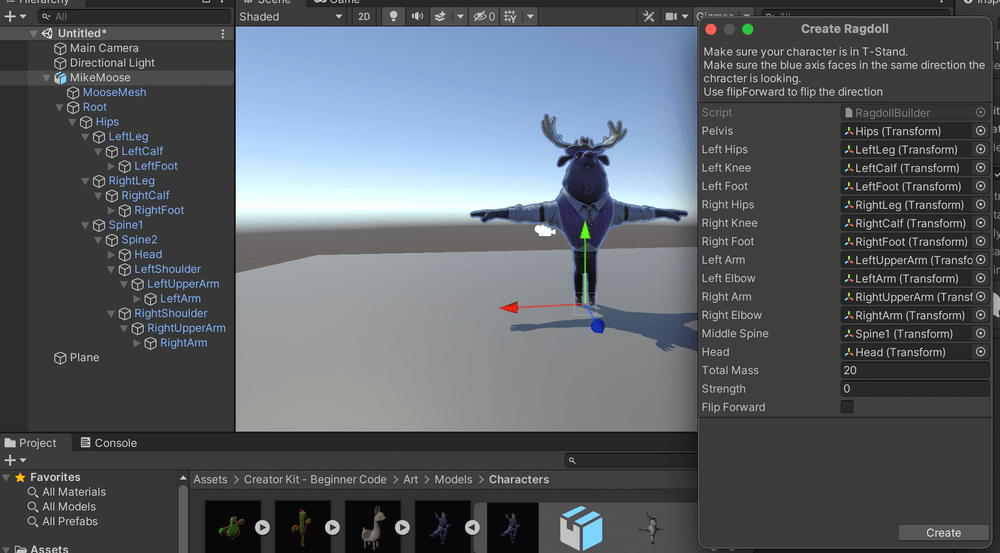
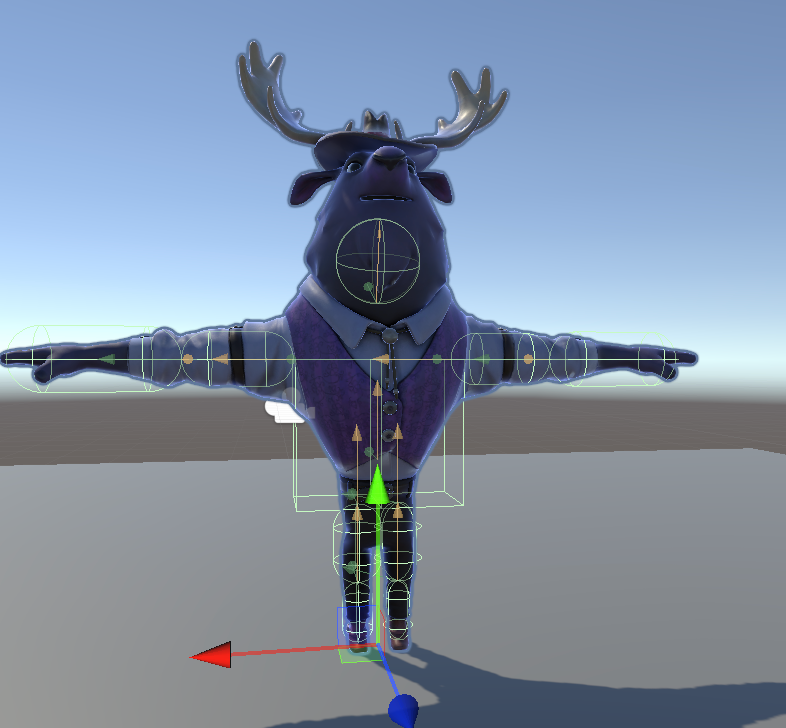
The above picture shows that the head collider is not accurate so we need to first adjust that so that head does not go below ground. After all the above steps are followed, your character is now physically simulated but the problem is when you add an extra collider in the main model, the character starts behaving in a weird way. We need to enable the main collider when we move and disable it when we want our rag doll to be active. Now let's see how we can do just that.
Initialization:
// colliders that needs to be enabled when not using ragdoll
public Collider[] colliderToEnable;
// rigidbody that is activated when not using ragdoll
Rigidbody rb;
// all colliders that are activated when using ragdoll
Collider[] allCollider;
// all the rigidbodies used by ragdoll
List < Rigidbody > ragdollRigidBodies;
// animator used to controll different animation state of the character
public Animator anim;
/// <summary>
/// this stores reference of all the collider and attached rigidbodies used by ragdoll
/// </summary>
private void Init() {
allCollider = GetComponentsInChildren < Collider > (true); // get all the colliders that are attached
foreach(var collider in allCollider) {
if (collider.transform != transform) // if this is not parent transform
{
var rag_rb = collider.GetComponent < Rigidbody > (); // get attached rigidbody
if (rag_rb) {
ragdollRigidBodies.Add(rag_rb); // add to list
}
}
}
}
Above, we are taking the reference of all the colliders and rigidbody components used by the character. These should be enabled or disabled based on what we want our character to behave like. Use Init() call in Awake() to initialize all the references.
Enabling and Disabling
public void EnableRagdoll(bool enableRagdoll) {
anim.enabled = !enableRagdoll;
foreach(Collider item in allCollider) {
item.enabled = enableRagdoll; // enable all colliders if ragdoll is set to enabled
}
foreach(var ragdollRigidBody in ragdollRigidBodies) {
ragdollRigidBody.useGravity = enableRagdoll; // make rigidbody use gravity if ragdoll is active
ragdollRigidBody.isKinematic = !enableRagdoll; // enable or disable kinematic accordig to enableRagdoll variable
}
foreach(Collider item in colliderToEnable) {
item.enabled = !enableRagdoll; // flip the normal colliders active state
}
rb.useGravity = !enableRagdoll; // normal rigidbody dont use gravity when ragdoll is active
rb.isKinematic = enableRagdoll;
}
The above code will use stored references to enable or disable them based on what we want. If we want our character to have ragdoll we must disable the animator component as well as the main collider component used to collide in the environment and vice versa. For this to work now you just have to attach it to the main character and assign a reference to the animator and main colliders (not the one used by ragdoll). After everything has been set you can just call EnableRagdoll True to enable ragdoll and EnableRagdoll False to disable ragdoll.
Now you have successfully implemented ragdoll in your game. Thank you for taking the time to complete this tutorial with me.