Have you ever wondered how to add new features like scenes, 3D objects, images, etc., to your app without pushing updates to Play Store?
Let's say you have 10 scenes in a game. If you add all of these levels while building a game, it increases the downloadable .apk
file size. The large file size of the game might repulse new players and make it harder to push small updates. So, how do you attract these players and reduce the file size of your app?
The solution is to add only a few scenes and assets to the base app, and then leave the remaining assets as content that can be downloaded whenever they are required. This process can be implemented through AssetBundles.
Using AssetBundles
Step 1: Building the AssetBundle
First, we create the asset bundle file of the gameObjects
that we wish to download later in the game.
Assets > Editor
folder.Do not try to attach this script to any gameObject
in the editor, simply place it inside the Editor folder.
public class CreateAssetBundles
{
[MenuItem("Assets/Build AssetBundles")]
static void BuildAllAssetBundles()
{
Debug.Log("Building bundled asset");
try
{
string assetBundleDirectory = "Assets/StreamingAssets";
if (!Directory.Exists(Application.streamingAssetsPath))
{
Directory.CreateDirectory(assetBundleDirectory);
}
BuildPipeline.BuildAssetBundles(assetBundleDirectory, BuildAssetBundleOptions.None,
EditorUserBuildSettings.activeBuildTarget);
AssetDatabase.Refresh();
}
catch (Exception e)
{
Debug.Log(" failed to create bundled assets" + e);
throw;
}
}
}
We will then define the assets we create as AssetBundles
. You can create Asset Bundles that contain prefabs, images, or scenes in particular by defining their AssetBundle
type. For example, we create a sceneBundle
for scenes, a prefabsBundle
for prefabs, an imageBundle
for images, and so on and so forth.
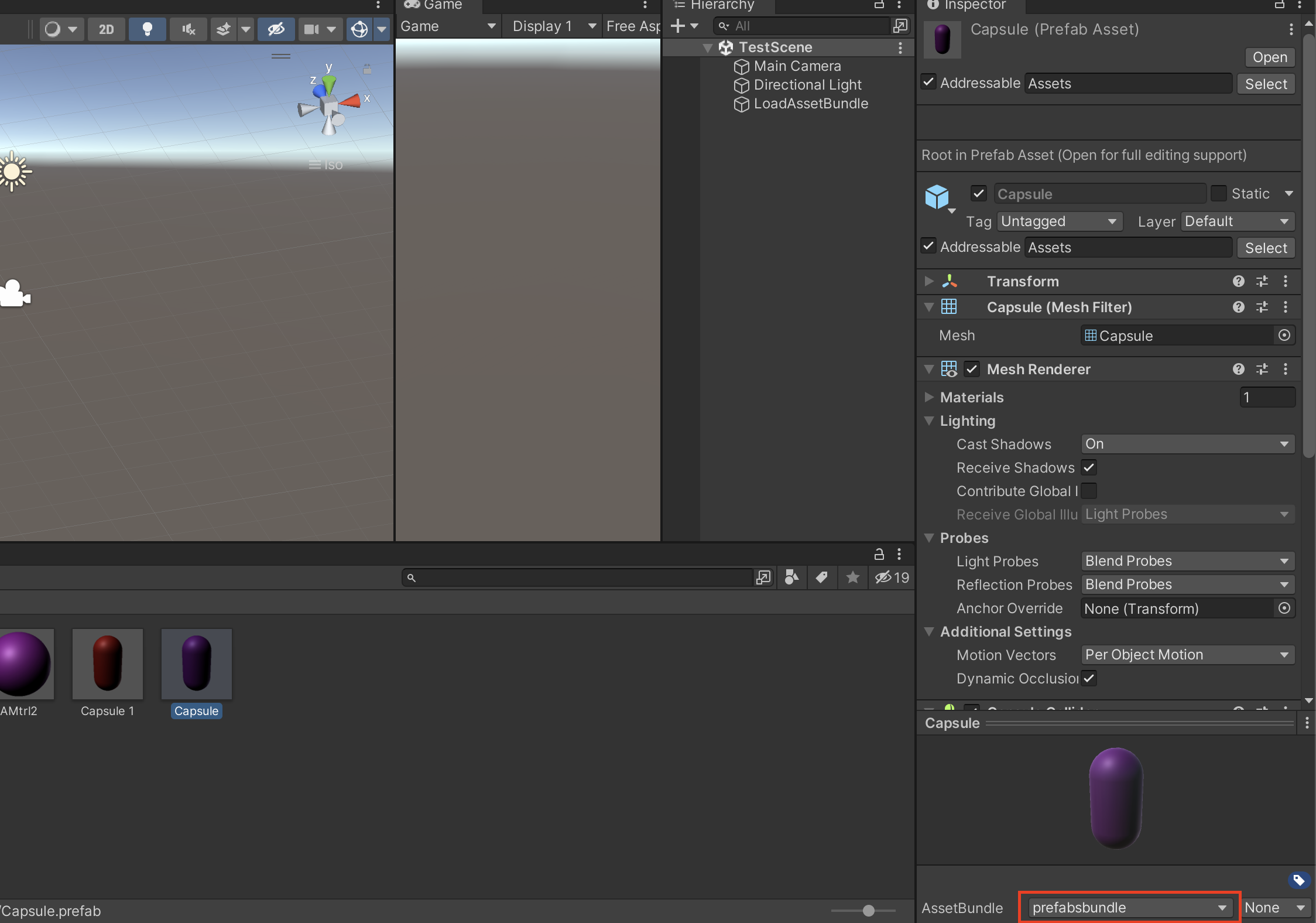
You can assign the particular AssetBundle
type through the drop-down menu of each asset. Open the menu, then click Create New
and choose whichever Bundle fits best. Do this for all gameObjects
to create a full Asset Bundle.
Now go to Unity Editor and Assets -> Build AssetBundles
.
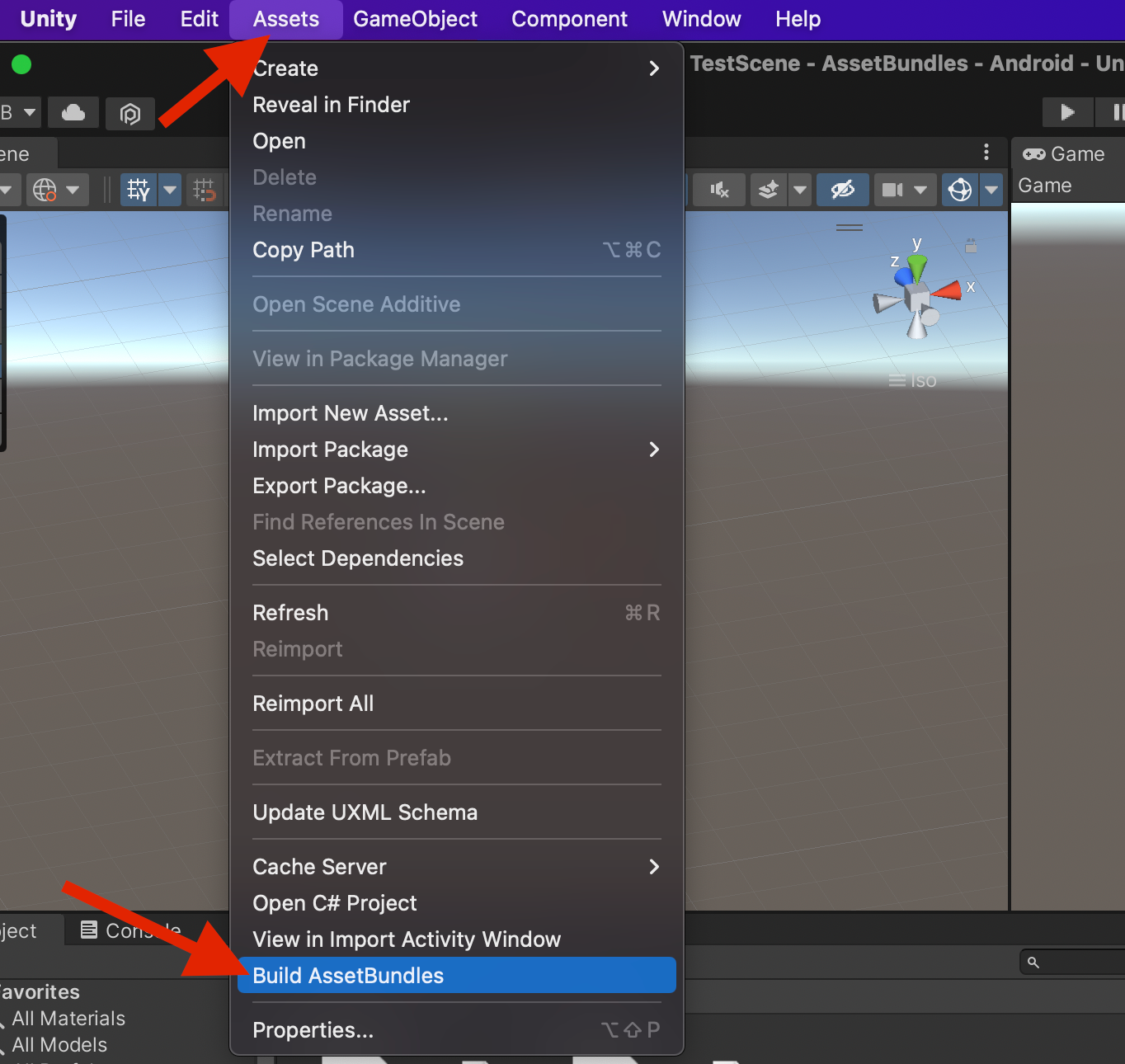
The Asset Bundle files will be created in the StreamingAssets
folder.
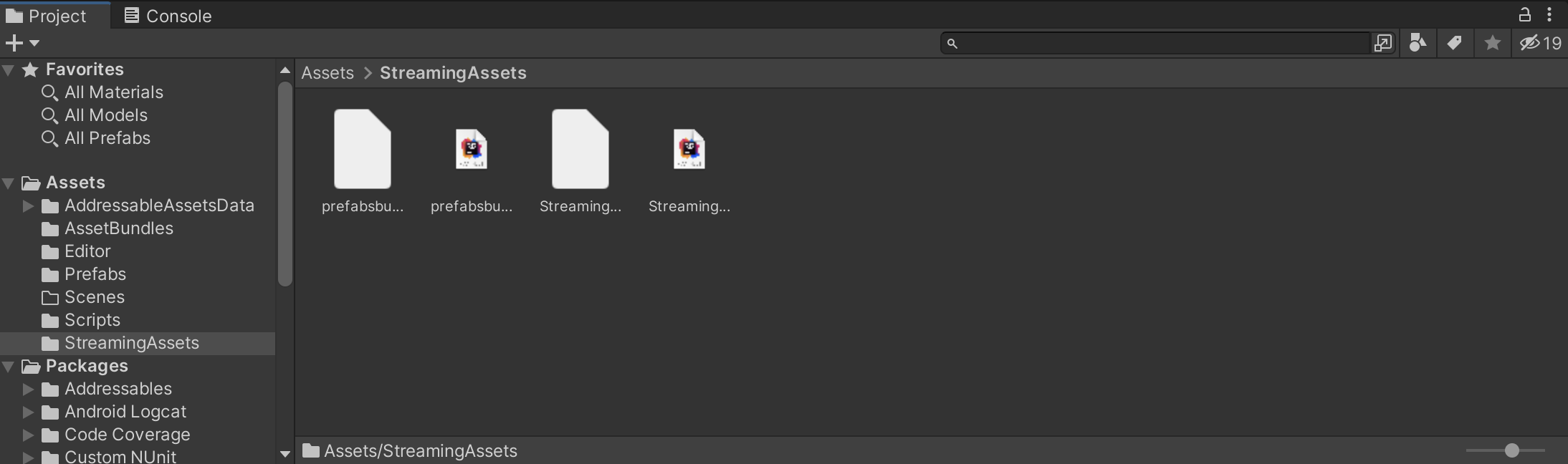
Step 2: Adding the AssetBundle to a Server
Now the remaining task is to place the Bundle files anywhere you like so you can download and use them according to your need. For each AssetBundle
, you should see two types of files being generated in your StreamingAssets
folder.
1) An extensionless file. This is the AssetBundle
file to download and use.
2) A file with a .manifest
extension. This contains info about the Asset Bundle's hash128, crcNumber, version, etc.
For testing purposes, place the extensionless assetBundle
file in a Google Drive.
Get the link of the file from Drive and convert it to a direct link. In my case, I used GoogleDriveDirectLinkGenereator. You can delete the created asset bundles in Assets > StreamingAssets
after you learn how to directly load scenes, images, and scenes from the link.
Step 3: Fetching AssetBundles
You are now all set up to fetch the Bundle files from your server and use them in your game. All you will need is this code.
IEnumerator FetchGameObjectFromServer(string url,string manifestFileName,uint crcR,Hash128 hashR)
{
//Get from generated manifest file of assetbundle.
uint crcNumber = crcR;
//Get from generated manifest file of assetbundle.
Hash128 hashCode = hashR;
UnityWebRequest webrequest =
UnityWebRequestAssetBundle.GetAssetBundle(url, new CachedAssetBundle(manifestFileName, hashCode), crcNumber);
webrequest.SendWebRequest();
while (!webrequest.isDone)
{
Debug.Log(webrequest.downloadProgress);
}
AssetBundle assetBundle = DownloadHandlerAssetBundle.GetContent(webrequest);
yield return assetBundle;
if (assetBundle == null)
yield break;
//Gets name of all the assets in that assetBundle.
string[] allAssetNames = assetBundle.GetAllAssetNames();
Debug.Log(allAssetNames.Length +"objects inside prefab bundle");
foreach (string gameObjectsName in allAssetNames)
{
string gameObjectName = Path.GetFileNameWithoutExtension(gameObjectsName).ToString();
GameObject objectFound = assetBundle.LoadAsset(gameObjectName) as GameObject;
Instantiate(objectFound);
}
assetBundle.Unload(false);
yield return null;
}
void Start()
{
StartCoroutine(FetchGameObjectFromServer(prefabBundleUrl,prefabsBundleName,a,b));
}
Attach this script to an empty gameObject
in the Editor and run the game. Voila, you will see your assets in the scene without them being present in your local device.
Conclusion
Remember to delete the created assetbundles
, in Assets > StreamingAssets
after you have loaded them from your server through this method. You will realize your .apk
download size has been reduced considerably, allowing for faster downloads and smaller updates.
AssetBundles are an easy way to make your applications more publishable online. I hope this tutorial gave you enough knowledge to begin using them in your work. If you have any queries, feel free to leave them in the comments below!