Unity has a very powerful animation and animator system, but those tools can have limited usage restrictions in some cases. These limitations are most apparent when you need to record animations in Unity's play mode.
Imagine you are creating a third-person game where the player can equip multiple guns, which means each gun can have its own hand pose. There are multiple approaches to record animations for each gun: from dragging and dropping the guns in your scene with the player to attaching every gun to the player and recording each gun animation.
However, there is a simple way to create animation clips. In this blog, we will use a handy Unity API GameObjectRecorder to create a simple yet powerful system that will help us record our animations in runtime and save them.
How I Got Here
For the purposes of this blog, I will be using my WIP third-person shooter Unity project. I have already implemented a system in my game that allows players to equip certain weapons and move/shoot with them.
While working on this project, I faced an issue when dealing with multiple guns with different unique meshes. I had to figure out a way to create exclusive hand pose animations respective to the gun designs without breaking the game because all of the guns shared a system that handled player and weapon interaction.
During my research, I found out about Unity's GameObjectRecorder API, which let me create and record animations at runtime, which was exactly what I needed.
So, starting off, you can see a character movement and weapon equip module I have created.
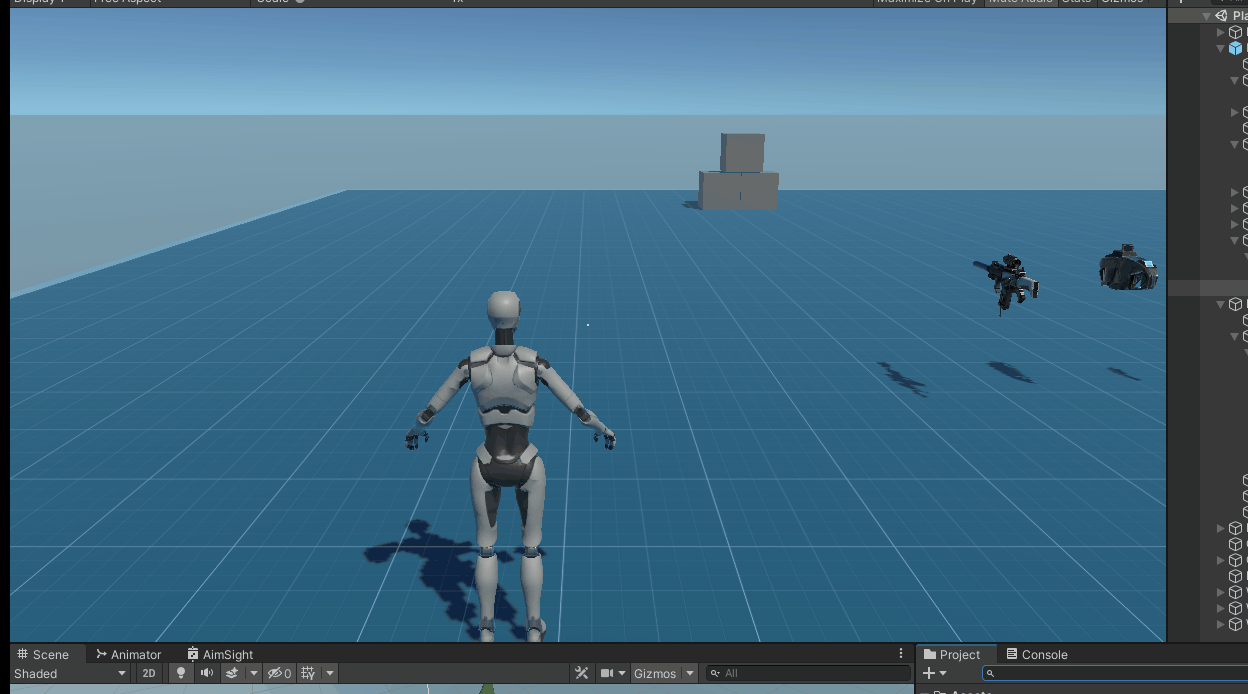
In the video, you can see each of those 3 guns have their own hand pose. That is our final goal.
We will divide the whole process into two parts :
- Scripting the Playmode Recorder
- Setting Up Hand Rig and Saving
Step 1: Scripting the Play Mode Recorder
We begin our process by scripting the play mode recorder. The script will be very simple; we will first use the GameObject recorder component and create a new GameObjectRecorder passing in our current GameObject; then, we will be binding the components we want to record.
In my case, I need to record the positions of my gun's parent object and the left and right hand grips that are constraints for the hand animation rigging of our character. As we just need the pose of our hand grips for our rig, we can take a snapshot of all the bindings in 0f
second intervals.
After that, we can save the recording to the clip we want using the SaveToClip()
function and pass it in our animation clip. To ensure that the animation is properly saved, we will have to save the assets to our unity assets database.
The context menu attribute we created above the method lets us call the method in the unity editor through the respective script's context menu.
[ContextMenu("Save weapon pose")]
void SaveWeaponPose()
{
GameObjectRecorder recorder = new GameObjectRecorder(gameObject);
recorder.BindComponentsOfType<Transform>(weaponParent.gameObject,false);
recorder.BindComponentsOfType<Transform>(weaponLeftGrip.gameObject,false);
recorder.BindComponentsOfType<Transform>(weaponRightGrip.gameObject,false);
recorder.TakeSnapshot(0.0f);
recorder.SaveToClip(weapon.weaponAnimation);
UnityEditor.AssetDatabase.SaveAssets();
}
Step 2: Setting Up Hand Rig and Saving
Once we have created the script, we can get into play mode and pick a gun we want to create a pose for.
In the video below, you can see I am moving the left and right grip of the character's rig to position the hand pose respective to the gun. After we tune the hands' positions, we can get to the script where we have created the Save Weapon Pose
function and click on its context menu.
It will show us a drop-down saying Save Weapon Pose
. Pressing it will save our gun's pose to the animation clip the weapon is referencing.
Close Unity editor and get back into play mode. If you go to the pistol and pick it up, you can see it has adapted the previous weapon pose you edited and saved in the play mode instead of using the default hand pose.
This was a simple usage of the GameObject recorder. The GameObject recorder can come in handy in other situations, like capturing real-time hand animations, recording physics-based animations, etc.
Thank you for reading and learning with me. Please leave comments if you have any regarding this article. Also, check out TheKiwiCoder, who was one of the major source for the blog.