Minio is a well-known, open-source object storage server compatible with the Amazon S3 API. This makes it easy for developers to use Minio in their applications to store and retrieve data from S3-compatible storage services.
Use Cases of Minio
One common use case for Minio is as a data storage solution for applications that needs to store large amounts of data. For example, Minio can store user-generated content such as photos and videos for applications like social media platforms or online marketplaces.
Another use case for Minio is a data storage solution for applications that store data in different locations or move their data between different storage services. For example, Minio can be used to store data in multiple cloud providers or to store data on-premises and in the cloud.
Minio is also often used as a data storage solution for applications that need to ensure data security. It offers strong security features, such as server-side encryption and support for multiple authentication methods, making it a good choice for applications that need to protect sensitive data.
Minio Scalability
One of the ways that Minio achieves scalability is through its distributed architecture. Minio is built on top of a distributed object storage system, which allows it to distribute data and requests across multiple nodes. This enables Minio to scale horizontally, enabling it to easily add more nodes to support more data and concurrent requests.
Minio also achieves scalability through its use of erasure coding. Erasure coding is a data storage technique that allows Minio to store data more efficiently and with less redundancy. It can store more data on each node and support more concurrent requests.
Besides, Minio offers various other features that help with scalability. Features include support for multi-tenancy, which allows multiple users or applications to share the same Minio instance, and support for cloud-native deployment models, such as containers and Kubernetes.
Overall, Minio is a highly scalable object storage solution that can easily handle large amounts of data and support high levels of concurrency. This makes it a good choice for applications that store and manage large amounts of data.
Installing Minio Using Docker
To install and run the Minio server in Docker, we will first need to install Docker on our system. Once Docker is installed, we can use the docker
command to pull the Minio server image from the Docker Hub registry.
Run the following command to pull the Minio server image from the Docker Hub in the console:
mkdir -p ~/minio/storage
docker run \
-p 9000:9000 \
-p 9090:9090 \
--name miniostorage \
-e "MINIO_ROOT_USER=your-username" \
-e "MINIO_ROOT_PASSWORD=your-password" \
-v ~/minio/storage:/storage \
quay.io/minio/minio server /data --console-address ":9090"
This will download the latest Minio server image from the Docker Hub, save it to your local Docker image repository and run it in the background.
Generating Access and Secret Keys for Minio
To generate access and secret keys for Minio, you can use the minio
command-line tool or the dashboard using the username and password we passed as environment variables inside the Docker of the Minio server.
Firstly, log in to the Minio server using the credentials we configured while installing the Minio server.
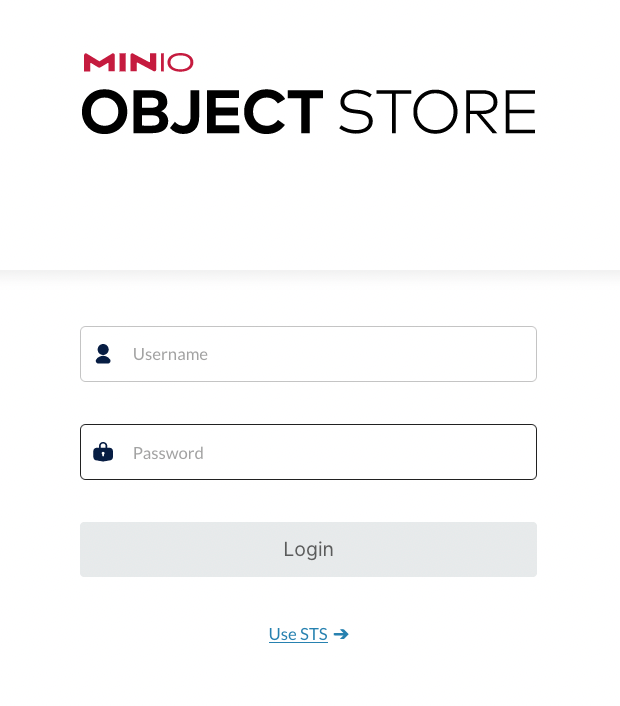
Secondly, after logging in, the dashboard will pop up. For now, we want to connect the client to the Minio server. So let's create access keys.
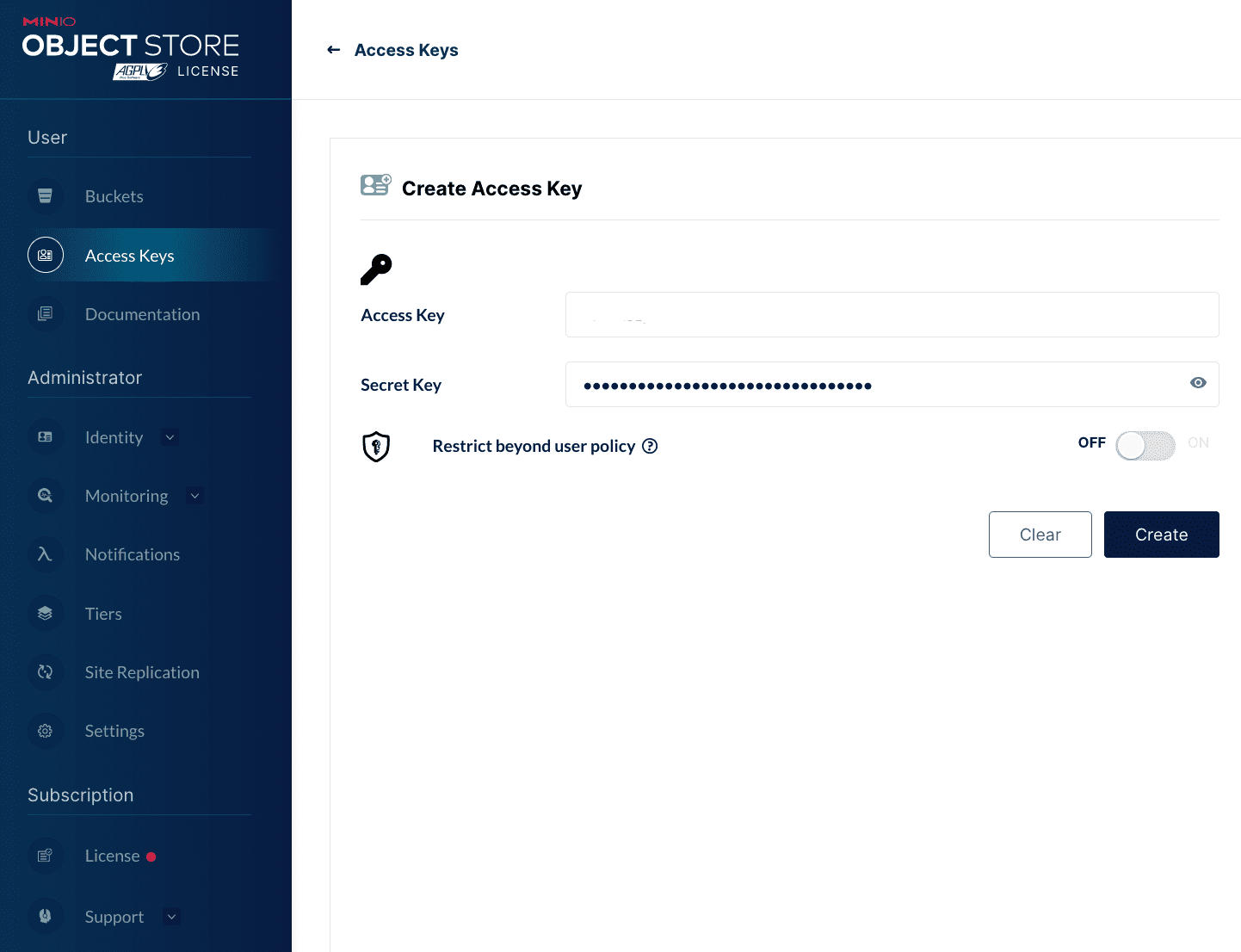
This code will generate new access and secret keys and save them for authentication. Those access and secret keys can be used to authenticate with the Minio server.
Connecting the Client to the Minio Server
To use Minio in Node.js, you first need to install the Minio package from npm. To install the Minio package, run the following command:
$ npm install minio
Performing Operations using Minio Package
Here is an example of how you might use the minio package to perform various operations on a Minio server in Node.js:
const Minio = require('minio')
const client = new Minio.Client({
endPoint: 'your-endpoint',
accessKey: 'your-access-key',
secretKey: 'your-secret-key'
})
Create a Bucket
// Create a new bucket
client.makeBucket('my-bucket', 'us-east-1', (err) => {
if (err) throw err;
console.log('Bucket created successfully');
})
List All Buckets
// List all buckets
client.listBuckets((err, buckets) => {
if (err) throw err;
console.log('Buckets:', buckets);
})
Upload an Object to a Bucket
// Upload an object
const object = 'hello-world.txt';
const data = Buffer.from('Hello, world!');
client.putObject('my-bucket', object, data, (err, etag) => {
if (err) throw err;
console.log(`Object "${object}" uploaded successfully. ETag: ${etag}`);
})
Download an Object
client.getObject(bucketName, object, (err, data) => {
if (err) throw err;
console.log(`Object "${object}" downloaded successfully. Data: ${data}`);
})
Delete an Object
// Delete an object
client.removeObject('my-bucket', object, (err) => {
if (err) throw err;
console.log(`Object "${object}" deleted successfully`);
})
In this example, we used the minio
package to create a new Minio client and connect to a Minio server. We then use the client to perform various operations on the server, such as creating a bucket, listing all buckets, uploading and downloading an object, and deleting an object.
In Conclusion,
Minio is a powerful and versatile object storage solution compatible with the Amazon S3 API. It is easy to use and customize and offers high scalability and reliability.
Minio is often used in Docker environments, as it can be easily deployed and run in a Docker container. It is also easy to integrate with other technologies and frameworks, such as NestJS and other languages like Go, Python, etc.
Minio offers various features and tools that make it a good choice for applications that store and manage large amounts of data. These include support for multi-tenancy, erasure coding, and cloud-native deployment models.
Overall, Minio is a valuable tool for developers and organizations that need a scalable, reliable, and easy-to-use object storage solution.
Hope you found this article helpful. Please subscribe and leave a comment if any queries.