Slicing can be confusing if you are new to it. With enough practice, you can understand its logic well and perform efficiently. So, this blog will explain the slicing concept in Python as simply as possible.
The basic concept of slicing is the same in most programming languages, but the syntax and specifics may differ slightly. I am familiar with Python, so I'll use it as the programming base to explain slicing.
Understanding Index
Before starting with slicing, you must have a concept of an index. Simply understand, the index is a position of a specific character or element in a list, tuple or string. There are two types of indexes – Positive Index and Negative Index.
In a positive index, the index value always starts with 0
(from the leftmost element) and ends with the total number of elements minus one (to the rightmost element). In a negative index, the index value always starts with -1
(from the rightmost element) and ends with the negative value of the total number of elements (to the leftmost element).
Remember that the one with the smaller absolute value is greater in negative numbers. For example, -1
is greater than -9
.
What is Slicing?
In general, slicing is used to extract a portion of an array, list, string, or other data structure and create a new data structure from it. The portion of the sliced data structure is specified by indices or range values that indicate the start and end points of the slice.
In some programming languages, such as C and C++, slicing is done manually by iterating through an array and copying the desired elements to a new array. In higher-level languages like Python, slicing is a built-in operation that can be performed using a slice notation.
Slicing is a useful technique for working with arrays and other data structures, as it enables you to extract only the portion of the data you need. This improves your code's efficiency and makes it easier to read and maintain.
Python slice()
Function
The slice()
function in Python is a built-in function that returns a slice object, which represents a slice of a sequence (such as a string, list, or tuple). The slice object can also be used as an argument to other built-in functions that support slicing, like list()
or str()
.
Syntax:
slice(stop)
slice(start, stop, step)
Parameters:
- start: The starting index of the slice is start, which is the first element you want to extract. By default,
start
is0
, which means the slice will start from the beginning of the sequence. - stop: The ending index of the slice, which is the first element that you don't want to include in the slice. By default,
stop
is the length of the sequence, which means the slice will include all the elements up to the end of the sequence. - step: The step value specifies the number of elements to skip between the sliced elements. By default,
step
is1
, which means that every element in the sequence will be included in the slice.
Examples of slice()
With one parameter
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
slice_obj = slice(6)
sliced_numbers = numbers[slice_obj]
print(sliced_numbers)
slice()
functionOutput:
[0, 1, 2, 3, 4, 5]
When you include only one parameter in slice()
, it is the stop parameter by default. In the above example, slice(6)
indicates that the slicing stops at the 6th index of the array, and the stop parameter value is not included.
start
value is 0
, so you need to count as 0
, 1
, 2
, 3
, 4
, 5
, 6
...With two parameters
Example 1:
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
slice_obj = slice(3,8)
sliced_numbers = numbers[slice_obj]
print(sliced_numbers)
slice()
functionOutput:
[3, 4, 5, 6, 7]
When you include two parameters in slice()
function, it is the start
and stop
parameters. In the above example, slice(3,8)
indicates that the slicing starts at the 3rd index of the array (the start index is always included) and stops at the 8th index (the stop index is never included).
Example 2:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
slice_obj = slice(3,8)
sliced_numbers = numbers[slice_obj]
print(sliced_numbers)
slice()
functionOutput:
[4, 5, 6, 7, 8]
To better understand this example, count the index as 0, 1, 2, 3,... Start from the 3rd index (number 4, included) and end at the 8th index (number 9, not included).
With three parameters
Example 1:
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
slice_obj = slice(1,7,2)
sliced_numbers = numbers[slice_obj]
print(sliced_numbers)
slice()
functionOutput:
[1, 3, 5]
Since the default value of the start
parameter in slicing starts with 0
, I tried to give an example in the same format. In the above example, I sliced the array- starting with the 1st index (number 1, included), which stops at the 7th index (number 7, not included) and jumps to the 2nd (1..3..5) element from every sliced element.
The text description might be confusing, so I drew a figure to explain the process.
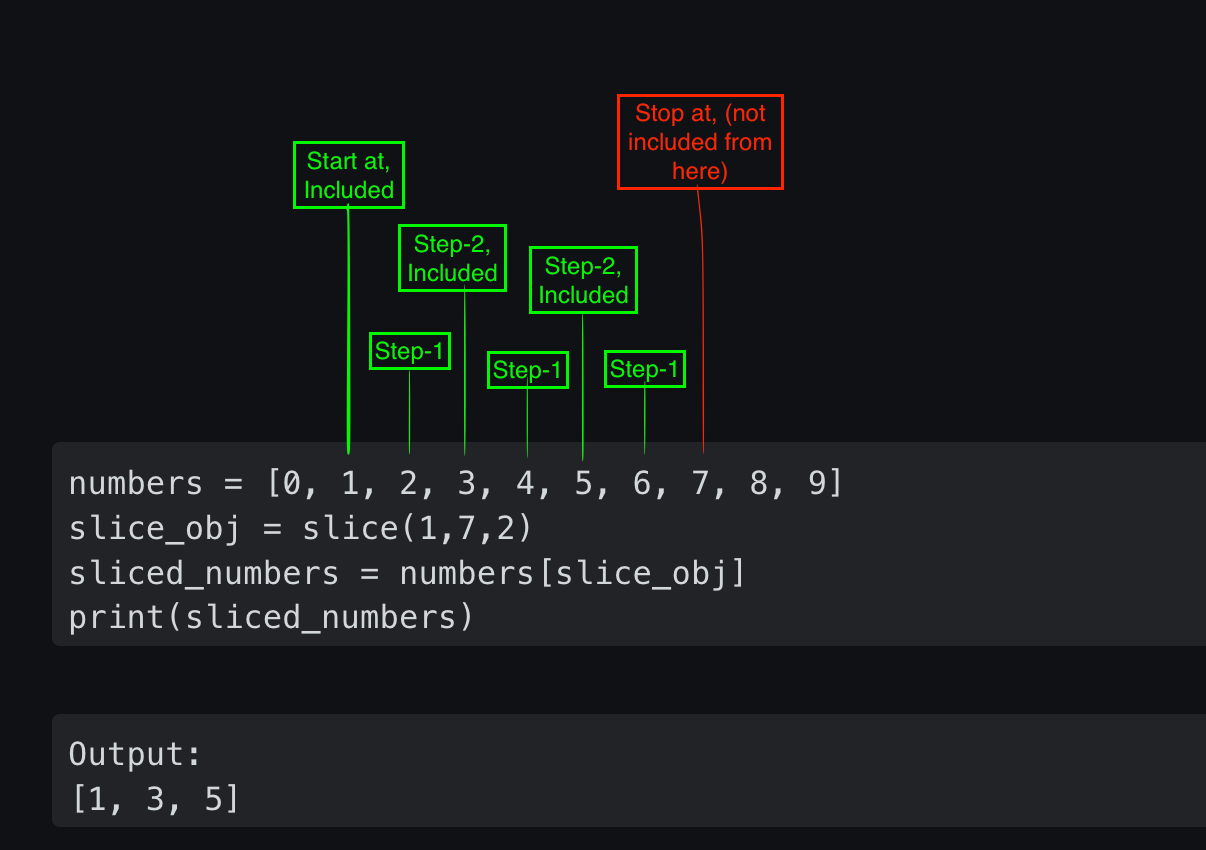
Remember, in this example, I have given the array the same as that of indexing so that it will be easier for you to understand.
Let me provide you with a different example anyways.
Example-2
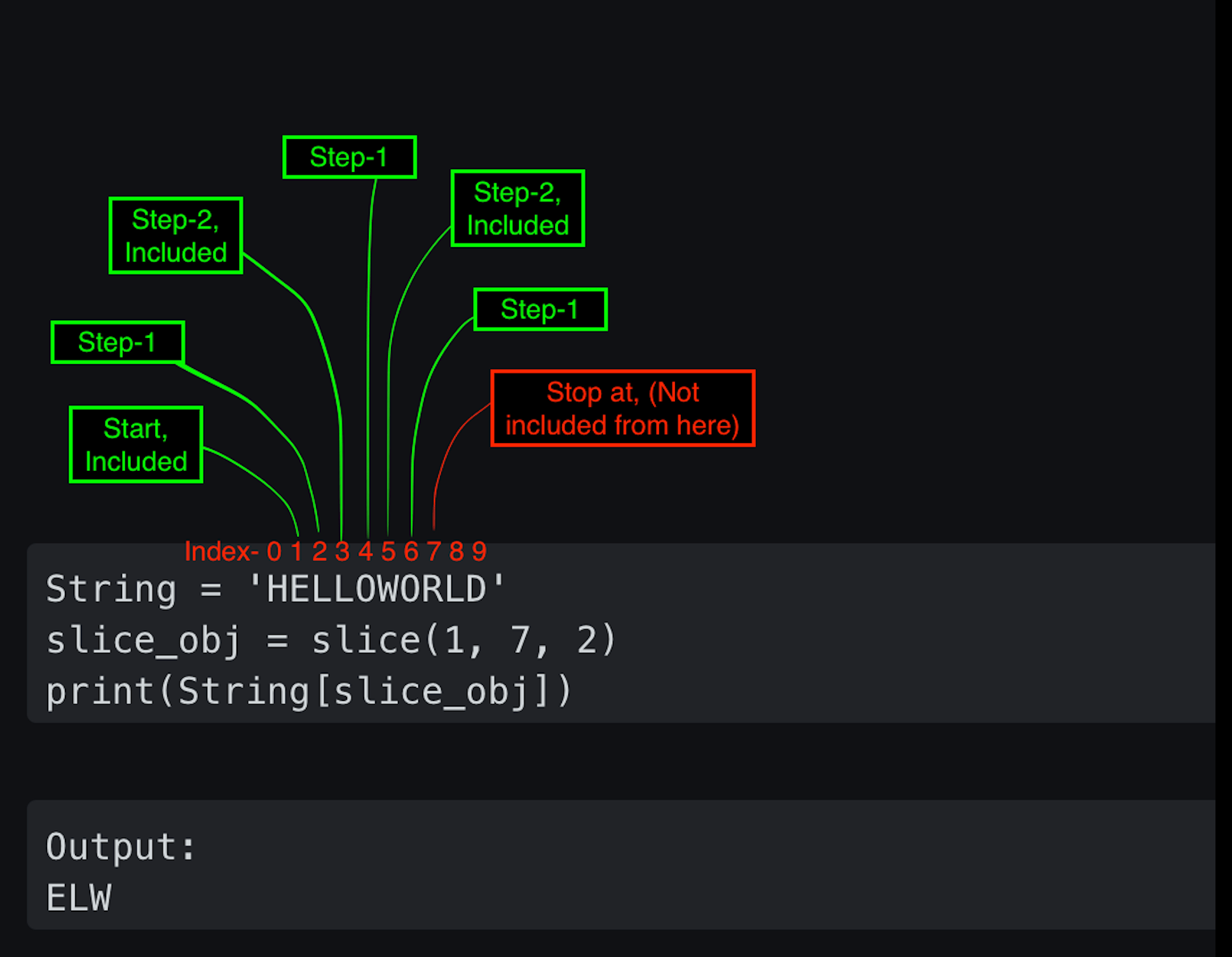
Using Negative Index
The slice()
function in Python also supports negative indexing. Remember that the start
parameter in negative indexing should always be the negation of a greater absolute number than that of the stop
parameter, and the step
parameter is always the positive value.
Example:
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
slice_obj = slice(-8, -2, 2)
sliced_numbers = numbers[slice_obj]
print(sliced_numbers)
slice()
function using a negative indexOutput:
[2, 4, 6]
The above example sliced the array starting at the -8th index (number 2, included), including every second element from the sliced element until the -2nd index (number 8, not included).
Explaining diagrammatically:
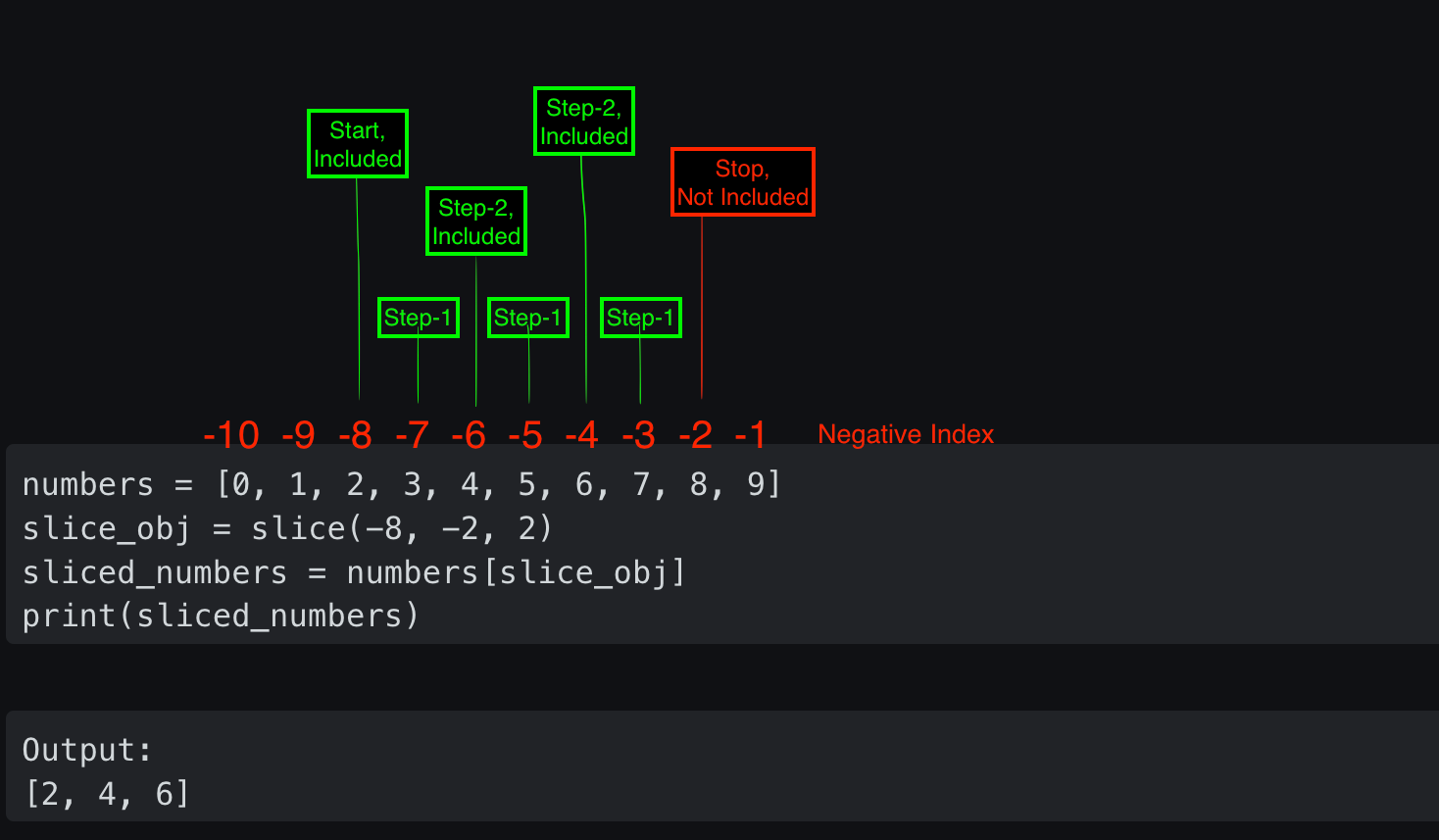
Lastly,
the start
, stop
and step
parameters logic in slice()
function can be quite confusing to grasp at first. My main purpose in writing this blog is to minimize the confusion regarding the same thing. If you are still confused, analyze the result multiple times to understand the logic. You can code the program any way you like following the coding rule and syntax per your programming language.
Subscribe! And if you still need some guidance on sorting out the parameters, let me know by commenting below.