If you have been trying to work with Flutter, you have already seen and heard about BuildContext
. Today we will deep dive into BuildContext
in Flutter with a widget tree.
A widget tree consists of an actual data structure built by the Flutter framework, which clearly shows the app structure with widgets and is used in developing an app.
Build Method for Widget Tree
We are building a widget tree via the build method in widget objects. Our Flutter projects consist of many widgets stored as a tree concept, having root, parent, child and siblings.
Whenever the build method returns more widgets used in programming, there are some specific points where the build function wants to return the same widgets.
It is expected from the method if we write the return type as follows:
Widget buildFunction(){
return Container(
color: Colors.blue,
child: const Text("Return any widget like , container , row ,column , sizedBox from here."),
);
}
From here, you can return any widgets from the buildFunction()
method. It accepts all kinds of widgets here. But if you change Line 1
with
Container buildFunction(){
...
The container is accepted for the above build method. It is because its return type clearly shows that it's a container. So from this method, any other widgets are not accepted.
Widget Tree
In Flutter, everything is a widget, and every widget follows a proper structure of a widget tree. For example, container, row, column, padding, size box, material widgets, scaffold, singleChildScrollView, button, customScrollView and many more. Through the widget tree, the parent and child communicate with each other.
Examples of Widget Tree:
...>MyApp
...>MaterialApp
...>Scaffold
...>AppBar, Row
...>Container, SizedBox, Row, Column
...> Text, Button, TextButton, TextFeild, Container , Expanded
My App – Root of the application
MaterialApp – Renders Scaffold
Scaffold – Renders AppBar and Row
Containers – Renders body portions of the applications like sizedbox, row, column, text, text button, etc.
BuildContext
In Flutter, every class and method contains @override
and build()
with the argument of BuildContext.
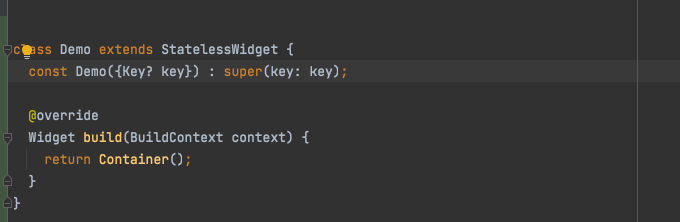
It presents a set of methods that can be used from
StatelessWidget.build
methods and methods on State objects.Examples of Build Methods
Some examples of build methods are:
Theme.of(context) – Nearest enclosing theme of the build that context.
Pop.of(Context) – Navigator context for navigating pages.
Builder.builder – Passing the BuildContext itself.
Scaffold.of(context) – Widgets function which holds scaffold.
Use the of()
Method
- To reference other widgets.
- Needed for inherited widgets.
- Tree for the next parent widget when passing the primary property.
- Showing the clear relation of
BuildContext
, if it is available or not.
@override
Widget build(context) {
return Text('Of method',
style:TextStyle(color: Theme.of(context).primaryColor),
);
}
When to Use BuildContext?
Every widget in a widget tree is in its own space. They have their own position, siblings, parent and child description. You can use any widget inside another. Before using widgets inside widgets, read their expected widgets accordingly.
In any case, widgets do not contain any information; we need BuildContext.
Why is BuildContext Needed?
BuildContext is needed for various purposes. Some of the major purposes of BuildContext are mentioned below:
- For locating the widget in the widget tree.
- Nested widgets (widget of widgets).
- Parent objects.
- For the state communication.
- For state management and data communication.
- Based on everywhere in context.
An Example of BuildContext
Here's a small example of using BuildContext, which you can try on your device with the widget's class.
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Build Context Example"),
center title: false,
),
body: SizedBox(),
floatingActionButton:
FloatingActionButton(onPressed: () => print(context.hashCode)),
);
}
BuildContext objects are the implementers called Element Objects. It is used to discourage the direct manipulation of elements.
More Details on BuildContext
Implementer – Element
Constructors – BuildContext()
Properties of Widgets
Method | Data Type | Description |
---|---|---|
debugDoingBuild | bool | used for currently updating the widgets or render tree, this option is read-only |
hashCode | int | inherited read-only hash code for the object |
owner | BuildOwner? | nullable and read-only context for managing the render pipeline of object |
runtimeType | Type | runtime type of widgets or functions or model |
size | Size? | size of render widgets |
widget | Widget | widgets used in finalrenderobject on UI |
Methods of Widgets
Method | Data Type | Description |
---|---|---|
dependOnInheritedElement | InheritedWidget | working on the base of ancestor's widget and rebuilding when ancestors change |
dependOnInheritedWidgetOfExactType | T? | runtime type of widgets or functions or model |
describeElement | DiagnosticsNode | descriptions of elements |
describeMissingAncestor | List | List of missing ancestors |
describeOwnershipChain | DiagnosticsNode | describes the ownership chain to the error report |
describeWidget | DiagnosticsNode | describe the details and working features of the widget |
dispatchNotification | void | bubble notification indicator at the context |
findAncestorRenderObjectOfType | T? | runtime type of RenderObjectOfType |
findAncestorStateOfType | T? | runtime type of StateOfType |
findAncestorWidgetOfExactType | T? | runtime type of WidgetOfExactType |
findRenderObject | RenderObject? | current render object which is created by itSelf |
findRootAncestorStateOfType | T? | runtime type ancestor's of given T type stateful widgets instance |
getElementForInheritedWidgetOfExactType | InheritedElement? | Type of concrete inheritedWidget subclass |
noSuchMethod | dynamic | dynamic type of method |
toString | string | converting the date to string through .toString methods provided by the framework |
visitAncestorElements | void | works for the call back return function, call back can not be null |
visitChildElements | void | children of the widgets or visitor |
Operators of Widgets
operator – bool
Things to Remember
- Before using BuildContext, remember that we must always ensure that the context of use on the method and the context needed for the method are the same or as expected.
- If the context does not match or you made some mistake, try and prevent such cases.
Conclusion
I hope you understood the widget tree, how it is collected, BuildContext and how it works with its methods, properties, implementations and operators. I hope you also got an idea of how BuildContext and widgets work in Flutter, similar to other languages like ReactJs and VueJs.
It all depends on our knowledge of the programming language and Flutter framework to build robust applications for production.
Thank you so much for reading. Please subscribe and leave a comment if you have any questions!