Node.js gives you the freedom to choose from various packages for serving web applications. Since there is no specific rule, you are free to use anything that meets your requirements. So, I started using express.js by default as it is the most popular package for web apps when working on such projects.
But then, I heard of fastify and how fast it was compared to its competitors. Obviously, I want my application to serve requests faster. I had to test if those rumors were true.
Setup
For the purpose of this speed test, I created a Node.js project with npm using:
mkdir testProject
cd testProject
npm init
Then I installed express@4.17.3 and fastify@3.27.4, both of which are the latest version at the time of writing.
npm install express fastify
I created the express.js and fastify.js files for testing purposes. They both contain a get request serving on the default route. To mimic a real scenario, I sent same json data in the response. The code for express.js is:
const express = require('express')()
const port = 3001
express.get('/', (req, res) => {
res.json({ hello: 'world' })
})
express.listen(port, () => console.log(`listening on port : `, port))
And the code for fastify.js is:
const fastify = require('fastify')()
const port = 3002
fastify.get('/', async (req, rep) => {
rep.send({ hello: 'world' })
})
fastify.listen(port).then(()=> console.log('listening on port : ', port))
Then, I executed both files in two terminals: using node express.js
for the code using express, and node fastify.js
for the code using fastify.
Testing
Now I need to test in a more realistic scenario, so I used "ab", the apache-benchmark tool to hit the API concurrently with requests.
After I got express and fastify running on port 3001 and 3002, respectively, I used the ab tool to examine the benchmarks for 100
requests with concurrency level 10
, mimicking 10 current users.
Running express.js test:
ab -n 100 -c 10 http://127.0.0.1:3001/
This resulted in:
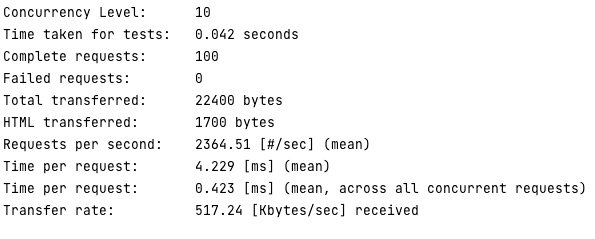
Running fastify.js test:
ab -n 100 -c 10 http://127.0.0.1:3002/
This resulted in
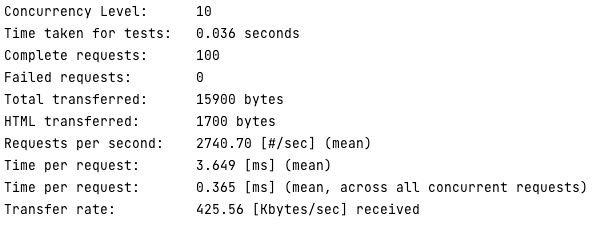
We can see a small performance gap from the results, as fastify was 0.006 seconds faster.
Extreme Testing
Then, I upgraded the requests to 10000
with concurrency level 100
. I ran the tests again.
Testing on express server resulted in:
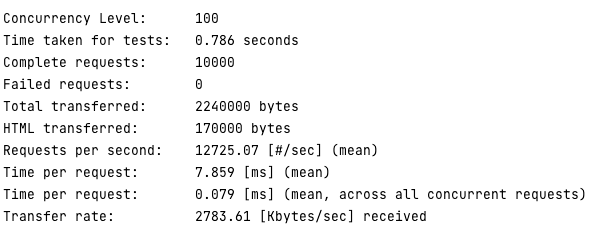
Testing on fastify server resulted in:
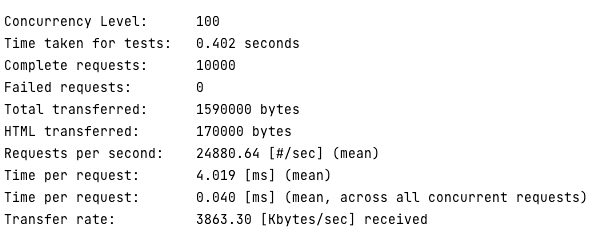
These numbers show a significant speed bump. Fastify was 0.384 seconds faster, which is almost half the time compared to express's 0.786-second result.
Conclusion
I was satisfied with what I saw, and started using fastify on my projects. Switching wasn't a great deal either. I am convinced that fastify is by far the faster web application framework.
If you want to test with further criteria, like post requests, more than 10000 requests, or more than 100 concurrency level, do it and respond in the comments below. Hope it helped!